
In one of our last posts we showed you how to make your swift code more sexy with a custom conditional assignment operator. Today, let’s look at another simple and cool improvement of dev’s daily life. It’s going to be about collections, and their well known method isEmpty
.
Let’s take a string, as an example, and check whether it’s empty or not.
let foo: String = "This string is not optional"
if foo.isEmpty {
// it's empty ?
}
It’s easy when the string is not optional, but what if we needed to check an optional string? We want to return true for both the empty string as well as for a nil value, don’t we?
By the way... Yes, string is a collection and you don’t have to use foo.count > 0, but I assume we all know that. ?
let foo: String? = "This string is soo optional ?"
// --- option 1 ---
if let bar = foo, !bar.isEmpty {
// it's not nil and also not empty
} else {
// it's nil or empty
}
// --- option 2 ---
if foo.map { $0.isEmpty } ?? true {
// it's nil or empty
}
// --- option 3 ---
if c == nil || c?.isEmpty == true {
// it's nil or empty
}
There are 3 different examples of how to achieve that and I think you can find dozens more, but all of these options have something in common - they’re badly readable, long and last but not least you have to think when you’re writing it and devs don’t like to think more than necessary. ?
It would be great to check an optional string like this…
let foo: String? = "This string is soo optional ?"
if foo.isEmpty {
// it's nil or empty ?
}
It is possible! All we need is to remember that Optional is enum, with two cases - some and none. ☝️ So we can create an extension on Optional where the type is Collection and implement new isEmpty
method.
extension Optional where Wrapped: Collection {
/// Return `true` if `self` is empty or nil
public var isEmpty: Bool {
switch self {
case .none:
return true
case .some(let value):
return value.isEmpty
}
}
}
Now you can check collections’ emptiness like a boss. ? Just directly copy-paste this snippet to your code or use our ACKategories, this and lot of other useful tricks are part of it, you will love it. ❤️
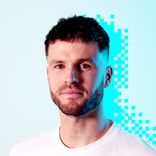